Intro to C
A quick introduction to the history and significance of C.

One of the most crucial instructions we can utilise while programming are conditionals. These allow for code to run only when some conditions are met. There are 2 main types of conditionals in C, the if statement and the while loop. The first one is the if statement, which is used to run code when certain requirements are met. This is incredibly important for developers as it allows them to create complex code that is able to perform actions based on conditions around it. An application for this could be in a game, someone needs to choose the correct item to unlock a secret area, and if the player does not choose the right item they do not get access. If the condition is not met, the code inside of the if statement is not run.
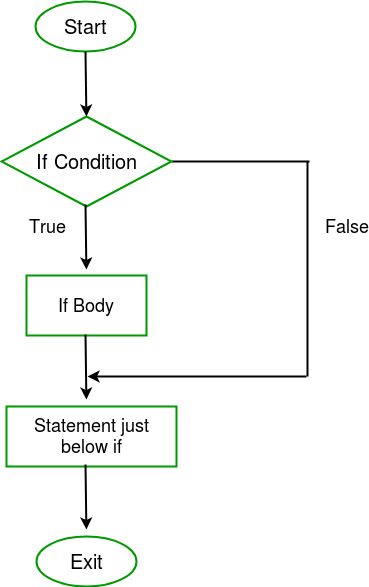
To actually write an if statement, we use the following syntax:
#include <stdio.h>
int main(){
if (//condition){
//code if condition is met
}
}
The amount of curly brackets may be a little confusing, however there are two sets of curly braces, the one inside is encapsulating the code that will be run if the condition of the if statement is met. Let's take a look at an example of an if statement in action:
#include <stdio.h>
x = 7;
y = 2;
int main(){
if (x - y == 5){
printf("x - y is equal to 5!";)
}
else if(x == 2){
printf("x is equal to 2!");
}
else{
printf("x - y is not equal to 5!");
}
}
In this example, we have declared two variables, x and y. In the conditional, if x - y = 5, the computer will print that x - y is equal to 5. Next, we have the else if component of the loop, which is able to provide another condition in the loop which will run seperate code, only if the code from the initial if statement is false. However, there is another part of this code that is new, which is the else statement. The section that is encapsulated by this else statement is run if the condition in the initial if statement AND if else statements is false. This is quite powerful as it allows for catchment of any other conditions that are not met by the initial if statement.
Following, we have the while loop. This allows developers to continuosly run code until a condition is met. This is important as if a developer would like one part of their code to run multiple times, and an unspecified amount of times, they can use a while loop. To write a while loop, we can write:
#include <stdio.h>
x = 0;
int main(){
while (x < 5){
x++;
}
}
After this, we have the for loop. The for loop is used to run code a specific amount of times. This is important as if a developer would like to run a portion of their code many times, but a specific amount of times, they can use a for loop. To write a for loop, we can write:
#include <stdio.h>f
int main(){
for (int i = 0; i < 5; i++){
printf("I want this to print 5 times!");
}
}
The structure of this loop may be a little more complicated so let us break it down. Firstly, we declare that we are using a for loop, and then we put in brackets to declare the conditionals. To start, we can declare a counter variable, which will count up every time the loop runs. This is indicated by the section ("int i = 0;"). Next, we have the condition that the loop will run until the counter is no longer less than 5. After, i++ is simply telling the computer to add 1 to the counter every time the loop runs. Now that you have been introduced to conditionals, there are a plethora of possibilities.